LETS LEARN
DEFINED DATA TYPES (typedef)
STRUCTURES (struct)
UNIONS (union)
ENUMERATION (enum)
ASSIGNMENT
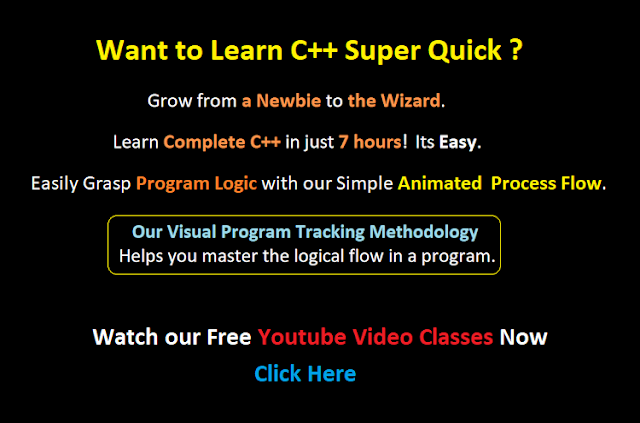
DEFINED DATA TYPES (typedef)
We can create duplicate names for the data types (int, char, etc). Such a duplicate name is called alias
data type. They are based on already
existing data types and can be used anywhere in the program to define data variables.
In C++ we can do this using the keyword typedef, whose format is:
typedef existing_type
new_type_name ;
where existing_type is the already available data type (like int, char etc) and new_type_name is the duplicate name for the
existing data type. For example:
typedef char C;
typedef
unsigned int WORD;
typedef char*
pChar;
typedef char
field[50];
In above example, we have 4 User Defined Data Types: C, WORD,
pChar and field … each of them being aliases for char, unsigned int, char* and char[50] respectively. We can
perfectly use them in declarations lateron as any other valid type:
C char1, char2, *ptc1;
WORD myWord;
pChar ptc2;
field studentName;
typedef does not create different types. It creates synonyms (copies) of existing types. typedef can be useful if a type you want to use has a name that is too long or confusing and is frequently used within a program. It is also useful to define types when it is possible that we will need to change the type in later versions of our program.
STRUCTURES
Structure is a user defined, composite data type that is used to group the data having different data types. We have already learned how groups of sequential data can be used in C++ using arrays and pointers, where, we store values of the same data type. But if we need to store a set of values having different data types, we need to use the Structures.
Structure is a collection
of data elements grouped together under one name. These data elements, known as members, can have different data types and different memory sizes. Data structures
are declared in C++ using the struct keyword
and the syntax is:
struct
structure_name
{
member_type1 member_name1;
member_type2 member_name2;
member_type3 member_name3;
. . . . . . . . . .
. . . . . .
. . . .
}
object_names;
where structure_name is user assigned name
of the structure, object_name can be a set of valid
identifiers that can be used to access this structure. Within curly braces are
data members, each one is specified
with a type and a valid identifier as its name. The first
thing we have to know is that a structure creates a new type.
Once a structure is declared, a new type
with the identifier specified as structure_name
is created and can be used in the rest of the program as if it was any other data-type.
For example:
struct product
{
int weight;
float price;
} ;
product apple;
product banana, melon;
We have first declared a structure
type called product with two data members: weight and
price, each of a different data
type. We have then used product as a structure type to declare three
objects: apple, banana and melon … just
like what we would have done with any other data type. Once declared, product has become a new valid type name like any other types (int, char, short etc.,) and from now
onwards, using this compound new type (product), we are able to declare objects (variables like apple,
banana and melon).
Right at the end of the struct declaration, and before the ending semicolon, we can use the optional field object_name to directly declare objects of the structure type. For example, we can also declare the structure objects apple, banana and melon at the moment we define the data structure type, like this:
struct product
{
int weight;
float price;
} apple, banana, melon;
Once we have declared our three objects of
a determined structure type (apple, banana and melon) we can operate directly
with their members. To do so, we use a dot
(.) inserted between the object_name and the member_name.
For example, we could operate with any of
these elements as if they were standard variables of their respective types:
apple.weight
apple.price
banana.weight
banana.price
melon.weight
melon.price
Let’s
see a real example where you can see how a structure type can be used in the
same way as fundamental types:
PROGRAM 45
Q. Write a program to accept and store a Movie Title and its Year of Release into a C++ Structure and then print it.
#include <iostream> using namespace std; struct movies { char title[50]; int year; }
data; main () { cout
<< "Enter Name of Film :
"; cin.getline(data.title, 50); cout
<< "Enter Year of Release : "; cin >> data.year; cout
<< "\nTitle :\t"<<data.title; cout
<< "\nYear
:\t"<<data.year; } |
OUTPUT
UNIONS
Union is a
user defined data type which uses the keyword union. It is similar to “Structure”
data type in declaration and use, but its functionality is very different. UNION allows storage of only one value
at-a-time. Hence, the memory allocated to store data in a union is that of the biggest data type declared within it. Union is used in certain cases where you
might need just one data (or set of data) to be used for processing some result,
from among multiple data types that can possibility be used for processing a result.
Syntax for declaration of union is:
union
union_name
{
member_type1 member_name1;
member_type2 member_name2;
member_type3 member_name3;
. . . . .
} object_names;
All elements of the union declaration occupy same physical space in memory. Its size is
the one with the greatest element in the declaration. For example:
union test
{
char c;
int i;
double d;
} mytypes;
defines three elements:
mytypes.c // a character of size 1 BYTE
mytypes.i // an integer of size 2 BYTES
mytypes.d // a double of size 8 BYTES
How the System Manages Memory Size in Union
In a Union, elements are of different data types. However,
the data type with maximum size is identified by C++ compiler. Here, data type
with maximum size is d of type
double. Hence, the memory size of this union named test will be 8 bytes (if test was a structure, memory size of test would
have been 1+2+8 = 11 Bytes).
Since all of the data in a union are
referring to the same location in memory (of size 8 Bytes), the modification of
one of the elements will affect the value of all of them. We cannot store
different values in them independent from each other. One of the uses a union can
have is to unite an elementary type with an array or structures of smaller
elements. For example look at this union:
union mix_t
{
long lg; // long integer of size 4 BYTES
struct
{
short sh1;
// short integer
of size 1 BYTE
short sh2; // short integer of size 1 BYTE
} s;
char c[3]; // character of size 3 BYTES
} mix;
Size
of this union is 4 Bytes. It defines three names that allow to access the same
group of 4 bytes: mix.lg, mix.s and mix.c and which we can use according to how we want to access these
bytes, as if they were a single long-type data (mix.lg), as if they
were two
short elements (mix.s.sh1, mix.s.sh2) or as an array of char elements (mix.c),
respectively. This union can be represented as:
The exact
alignment and order of the members of a union in memory is platform dependent.
Therefore be aware of possible portability issues with this type of use.
ENUMERATION (enum)
In C++, Enumeration is a user
defined data type that can
assign numeric values to a set of constants defined within it. Elements within the enumeration datatype can be referred to
by the numeric value or the named
constant. It uses the keyword enum when defining a new data type. Syntax of usage is:
enum enumeration_name
{
value1,
value2,
value3,
. . . . .
. . . . .
} object_names;
Here is an example:
enum colors_t
{
black, blue,
green=10, cyan, red, purple=20, yellow, white
} p ;
Notice that we do not use any basic data type (int, char…etc) in the declaration.
p is the object that is used to refer elements within the
enumeration data type.
Here, since black is declared as first value, it will be automatically assigned value 0, followed by blue = 1,
but next we have explicitly assigned green = 10, and so cyan will be 11 and red = 12,
again we have explicitly assigned purple = 20, and hence, yellow will be 21 and white = 22
Enumerations are "type compatible with numeric variables", so their constants are always assigned an integer numerical value internally. If it is not specified, the integer value assigned to first constant is 0, and the following ones are auto incremented by 1. We can also explicitly specify an integer value for any of the constant values and the next value automatically increases from there (in above example see how green is assigned explicit value of 10).
ASSIGNMENT:
Using
Structure Array, write a program to record the following fields of “N” students:
1.
Student
Name,
2.
Mark1,
3.
Mark2,
4.
Mark3,
5.
Total
Mark.
Display
a simple Mark List.
No comments:
Post a Comment