LETS LEARN
INTRODUCTION TO OOPs
Real World Example of an Object
Defining Class & Objects
FEATURES OF OBJECT ORIENTED MODEL
1. Encapsulation
2. Abstraction
3. Inheritance
4. Polymorphism
Static Polymorphism
Dynamic Polymorphism
ADVANTAGES OF OOPS
TOP LANGUAGES WITH OBJECT-ORIENTED FEATURES
CLASS
Access Specifiers in a Class
private, public, protected
Member Data
Member Functions
OBJECTS
Operations of an Object
STATIC MEMBERS IN A CLASS
(a) Static Data Members
(b) Static Member Functions
ARRAY OF OBJECTS
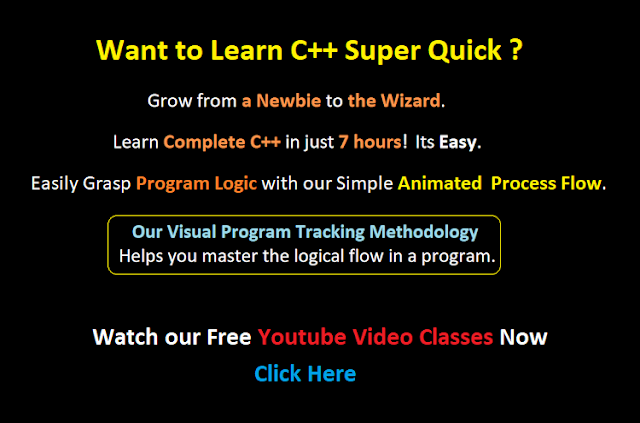
INTRODUCTION TO OOPs
Object Oriented Programming System (OOPs) is a software coding pattern, wherein you create OBJECTS (just like real-world objects) to perform certain tasks. These Objects have Form (data structure) and Roles (functional features).
Before creating an Object, we first have to create a prototype (or model) of this object. This model is called the CLASS. The CLASS comprises of member data fields and related functions that operate upon those data to achieve a task. The data within a CLASS are supposed to be modified only by functions within that CLASS.
These data and functions belonging to a specific CLASS are referred to as encapsulation. Encapsulation provides protection to the data & functions within a CLASS by preventing direct modification of the data from outside the CLASS. Based on the prototype model (CLASS), a block of memory space is reserved (allocated) to store data and functions of the CLASS.
The memory allocated space for a CLASS, is the OBJECT of that CLASS. The process of creating an OBJECT from a CLASS is known as "instantiating" a CLASS (or creating the instance of a CLASS).
In the earlier chapter you learned about Structures. Difference between a Structure and Class is that, the Structure has only data fields whereas a Class has both data fields and functions that operates upon those data fields. An Object is the actual memory allocated to an instance of the Class.
Real World Example of an Object
An
OBJECT in OOP can be compared to any object you see in the real world, having
certain Characteristics (giving it a form and shape or state) and Functionality (defining its behaviour). For example, considering your
mobile phone as an object, the following can be outlined.
Object : Mobile Phone
Characteristics : Screen,
Outer-Cover, Sim-Card, Battery, Mother-Board
Functionality : Making Calls, Sending Messages, Face Book Access etc.
In
OOPs, the Characteristics can be
equated to member Data, and the Functionality
can be equated to member Functions.
Definition of Class & Objects
CLASS |
A Class is the construct or template declaration for an Object. It
is the logical entity of a user defined type or data structure declared with keyword class having data and functions.
|
OBJECT |
An Object is the physical entity or
instance of a class that is created dynamically to store real time data
and execute certain functionality. This process is called instantiation.
|
FEATURES OF OBJECT ORIENTED MODEL
Encapsulation
is the process of wrapping (grouping) together of data and functions into a
single entity (the Class). Encapsulation
provides protection to the data & functions within a CLASS by preventing direct modification of the data from outside the
CLASS. It keeps
the implementation details private to an Object. Restricting access to the functions
and data, reduces coupling (data duplication), which in turn increases the data
reliability in large programs. Classes also encourage coherence (guarantee),
which means that a given class does one specific task. By increasing coherence,
a program becomes easier to understand, less complex, more simply organized,
and a further reduction in coupling.
2. Abstraction
3. Inheritance
Inheritance is the process of creating a Class (child Class) with characteristics (properties) and functionalities (behaviours) of an existing Class (parent Class). The newly created child class, not only inherits all capabilities of the parent class, but also adds up its own data and functions. The new class is called: derived class, child class, or subclass. The original class is called: base class, parent class, or superclass.
4. Polymorphism
Polymorphism is the ability of a single function to perform different tasks under different circumstances. There are two types of polymorphism: Static polymorphism & Dynamic polymorphism.
Static Polymorphism is exhibited
by overloaded functions (please
refer Chapter 8: Functions). Static polymorphism is the technique of overriding a function by providing
additional definitions with different numbers or types of parameters. The compiler matches the parameter list
to the appropriate function that is called in the program. (Remember how we had
used function polymorphism in the last chapter). Here you declare and define
multiple functions with same name
but different parameters. For
example, consider the following function declarations:
void add(int , int);
void add(float, float);
(i) Re-use of code. Linking of code to objects and explicit specification of relations between objects allows related objects to share code.
(ii) Ease of understanding. Structure of code and data structures in it can be setup to
closely mimic the generic application concepts and processes. High-level code
could make some sense even to a non-programmer.
TOP LANGUAGES WITH OBJECT-ORIENTED FEATURES
C++
C#
JAVA
PHP5
OBJECTIVE C
PYTHON
RUBY
VISUAL BASIC
CLASS
A Class is the construct or template declaration for an Object. It forms the basis for Object-Oriented programming. Class is used to define the nature of an object, and it is the basic unit of encapsulation in C++.
Classes are created using the keyword Class. A class declaration defines a new user-defined type that links code and data. It is an expanded concept of a data structure: instead of holding only data, it can hold both data and functions.
An Object is an instantiation of a class. In terms of variables, a class would be the type, and an object would be the variable. Classes are generally declared using the keyword class, with the following format:
class class_name { access_specifier
: members 1,2,3… ; access_specifier : members 4,5,6… ; ... }
object_names; |
Here class_name
is a valid identifier for the class, object_names
is an optional list of names for objects of this class. The body of the
declaration can contain data members
or function members with optional access specifiers.
ACCESS SPECIFIERS
Access Specifiers are used to set the access rights of data/function members
within a Class. An
access specifier uses any one
of the following three keywords: private,
public, protected.
private members of a class are only accessible by other members within the same class or from within friends of a class. This helps data hiding. Member Data and Member Functions can be declared under the private section by using the private keyword followed by a colon.
private: int p, q ;
public members of a class are accessible from anywhere where the object is visible. An application can use the private member-data of a class only through the member-functions that are declared as public. Member Data and Member Functions can be declared under the public section by using the public keyword followed by a colon.
public: int p, q ;
protected members of a class are accessible by members of the same class, by their friends and also by members of their derived classes. Member Data and Member Functions can be declared under the protected section by using the protected keyword followed by a colon.
protected: int p, q ;
Member Data within a Class, are the variables which determine the attributes of that class. Every single object is a separate entity and hence has separate copies of data members. The memory allocation of data members is done at the time of object creation.
Member Functions within a Class, are the methods by which a
class interacts with the outside world. Only one set of member functions are
created for all objects of same class. Each object uses the same member
functions with own values of data members. Memory allocation of member
functions is done at the time of class creation (function definition).
OBJECTS
Object in C++ refers to an instance of a
class. A class defines the characteristics (behaviour)
of its instances. The characteristic of
a class is defined in terms of data members
(state), member functions (methods or operations), and the visibility of these
members to other classes.
A class defines the behavior of possibly
many objects (instances). Objects are
usually referred to by references, which are aliases for an object. Each object of the class
that is created gets a copy of all the class data members, except for those
declared as static. All objects of a particular class share the member
functions for that class.
Operations of an Object
Operations
are defined by functions. Functions
may be either globally defined
(independently of object classes), or as
part of class definitions (member functions). C++ essentially supports
member functions that are within a single object class. However, friend
functions that are outside these classes, do have access to the internals of
instances of another class. Syntax to declare on object as variable:
class_name object_list;
Syntax to
declare an object with the class definition
class class_name
{
access_specifier_1:
member1;
access_specifier_2:
member2;
...
} object_list;
If not specified, all members of a Class are assigned with private access by default. Therefore, members that are declared before a class specifier, are automatically assigned with private access visibility. For example:
class CRectangle // class declaration
{
int x, y; // member-data declaration with visibility PRIVATE
public: // scope visibility
declaration
void setValue (int, int); // member-function
declaration with visibility PUBLIC
int area (void); // member-function
declaration with visibility PUBLIC
}
rect; // object
variable declaration
The above is declaration of a Class called CRectangle and its Object called rect. This class contains 4 members:
- 2 data-members of type int - x, y with private access (because private is the default access level)
- 2 member-functions - setValue(), area() with public access
For now, we have only included function declaration, not their definition. Remember that, a function can be defined inside the Class declaration, or outside the Class declaration as inline function using the scope resolution operator. An Object can also be declared outside Class declaration like this:
CRectangle
rect ;
NOTE: In the above example, CRectangle is the Class name (i.e., the type), whereas rect is an object (variable) of type CRectangle. Hence, Class Name (CRectangle) = Data Type Object Name (rect) = Variable It is similar to the following declaration: int a; where, int = Data Type a = Variable
|
After the
previous declarations of Class CRectangle and Object rect, we can call any public members of the object rect,
within the body of the program by using the object name followed by a dot (.) and then the name of the member
data/function. For example:
rect.setValue (3, 4);
myarea = rect.area();
The only members of rect that we cannot access from the body of our program outside the class are x and y, since they have private access and they can only be referred from within members of that same class.
PROGRAM 56
Q. Write a program to find the area of a rectangle using class and objects. Requirements: Declare two functions setValue(), area(). Define area() within the Class. Define setValue() outside the Class.
#include <iostream> using namespace std; /* Class declaration */ class CRectangle { int x, y; public: void setValue (int, int); /* Inline definition for member Function */ int area ()
{ return (x*y); } }; main() { CRectangle rect; rect.setValue (3, 4); cout << "Area of Rectangle =
" << rect.area(); } /* member Function
definition outside the Class */ void CRectangle :: setValue (int a, int b) { x =
a; y = b; } |
PROGRAM 57
Q. Using Class & Objects, write a program to find the area of 2 Rectangles.
(Requirements:
Use 2 functions setValue(), area(). Declare & Define both functions within the
Class. Instantiate values for first rectangle. Accept keyboard values for second
rectangle)
#include <iostream> using namespace std; class Rectangle { int x, y; public: void setValue (int a, int b) { x =
a; y =
b; } int area () { return
(x*y); } }; main () { Rectangle r1, r2; r1.setValue (3,4); int i, j; cout << "Enter Length of 2nd
Rectangle : "; cin >> i; cout <<
"Enter Breadth of 2nd Rectangle : "; cin >> j; r2.setValue (i,j); cout<< "\nArea of first
Rectangle = " <<r1.area()
<< " Units\n"; cout<<
"Area of second Rectangle = " <<r2.area() << "
Units"; } |
OUTPUT:
STATIC MEMBERS IN A CLASS
From
Program 57, let’s recall that for a Class named Rectangle, we had declared two Objects: r1 and r2. Data members x, y of r1 and x, y of r2 were stored as two different copies in the
computer’s memory. Any change in x, y of r1 would not affect x, y of r2. Imagine that, if x and y had only a single common location
in the memory, and accessible to r1 and r2, then x, y would be called the
static members. Any changes made to the static member in one object of a class, would affect all other objects (of the same class) accessing it.
Data/Function members of a Class, that acts as the single copy for all objects of that class and accessed from anywhere within a program, are called static members. A static member is shared by all objects of the class. Static members are both data members and member functions.
(a) Static Member: Data
Static Data Members share only one copy between all objects of the same class. It is automatically initialized when the first object is created. It’s visibility is only within the class but its life time is till the end of the program. The important point is that the static data members need to be defined outside the class. Syntax for declaring a static variable:
static data_type data_member_name; ( Eg: static int a; )
or
data_type static data_member_name; ( Eg: int static a; )PROGRAM 58
Q. Define a Class named “C” with 2 variables: Count1 (static int) and Count2 (int).
Use DisplayCount() to print Count1 and Count2. Instantiate 4 Objects of this Class and call
DisplayCount() of each Object.
#include<iostream> using namespace std; class C { private: static
int Count1; int
Count2=0; public: void
DisplayCount(); }; void C :: DisplayCount() { Count1++; Count2++; cout<<"Print
Count (Static) = "<<Count1<<"\t Print Count (Ordinary)
= "<<Count2<<endl; }
/* static variable is defined outside the class. Count1 = 0 is auto assigned */ int C :: Count1;
main() { C Obj1, Obj2, Obj3, Obj4;
Obj1.DisplayCount();
Obj2.DisplayCount();
Obj3.DisplayCount();
Obj4.DisplayCount();
} |
(b) Static Member: Functions
Static Member
Functions can access only other static members of the same class. Static
functions can be accessed through their class name without actually creating
the object. The keyword static needs to be given while declaring the
member function. Syntax for declaring a static member function:
static return_type function_name(argument_list);
OR
return_type static function_name(argument_list);
A static
function can also be called with the Class name if declared as public member. Syntax
for calling static member function using Class name:
class_name :: member_function_name (argument_list);
PROGRAM 59
Q. Define a Class named “C” with 1 variable: Count (static
int). Use static function DisplayCount() to print value of Count. Instantiate 4
Objects of this Class and call
DisplayCount() of each Object. Finally, call DisplayCount() with direct reference
to the Class.
#include<iostream> using namespace std; class C { private: static
int Count; public: static
void DisplayCount(); }; void C :: DisplayCount() { Count++; cout<<"Number
of times = "<<Count<<endl; }
/* static variable defined outside the class.
Count=1 is auto assigned */ int C::Count;
main() { C Obj1,
Obj2, Obj3, Obj4;
Obj1.DisplayCount(); Obj2.DisplayCount(); Obj3.DisplayCount(); Obj4.DisplayCount(); /* Function
called with class name */ C ::
DisplayCount();
} |
OUTPUT:
An array of Objects is a collection of several Object variables with a common name, occupying contiguous memory locations. Each element of an object array is referred by its index number. It provides simple & convenient way to store tabular information. An array can be 1 or 2 dimensional, depending upon the application requirement. When memory is allocated for an Object-Array, data members are separate for each array element but member functions are same and are shared among all objects.
Syntax for
declaring single dimension array:
class-name array-name[number-of-elements] ;
Syntax for
accessing public members of array elements:
array-name[element-number].member-name ;
PROGRAM 60
Q. Write a program using an Object-Array to input Employee number, Name and Salary of 2 Employees and display them.
#include<iostream> using namespace std; class Employee { int roll_no; char
n[20];
float salary;
public: void getData(); void
printData(); }; void Employee :: getData() { cout<<"Enter
Emp. Number : "; cin>>roll_no; cout<<"Enter
Emp. Name : "; cin>>n; cout<<"Enter
Salary : ";
cin>>salary; cout<<"\n"; } void Employee :: printData() { cout<<"Emp.
Name : "<<n; cout<<"\nEmp.
Number : "<<roll_no; cout<<"\nSalary : "<<salary; cout<<"\n\n"
; } main() { Employee e[2]; int i; cout<<"\nEnter
Data for 2 Employees \n\n";
for(i=0;i<2;i++) e[i].getData(); cout<<"Employee
Information \n\n";
for(i=0;i<2;i++) e[i].printData(); } |
OUTPUT:
No comments:
Post a Comment