LET’S LEARN
INTRODUCTION
CONDITIONAL CONTROL STRUCTURES
(a) if statement, if…else statement
(b) switch statement
(c) Conditional Operator / Ternary Operator
LOOPING (ITERATION) STRUCTURES
(a) while loop
(b) do-while loop
(c) for loop
Functional steps in the ‘for’ Loop
for loop without control expressions
for loop with control expressions separated by comma operator
JUMP STATEMENTS (UNCONDITIONAL CONTROL STRUCTURE)
(a) break Statement
(b) continue Statement
(c) goto Statement
(d) return Statement
CHAPTER EVALUATION EXERCISE
INTRODUCTION
In a program, the instructions are
not always limited to a sequential order. As per requirements and complexity of the functional need of a
program, you may need to make decisions or shift the control from one part of
the program to another. It may also be essential to repeat some areas of the
program code so as to achieve recurring tasks.
C++ provides conditional/unconditional control
statements which determines the order in which program statements are to be
executed.
C++
also provides looping statements
that controls the number of times an activity (a set of program statements) is to
be repeated.
Statements used in control structures and loops can either be a simple statement (a
simple instruction ending with a semicolon) or a compound statement (several instructions grouped in a block). In
the case that we want a compound statement, it must be enclosed between curly braces { } forming a block.
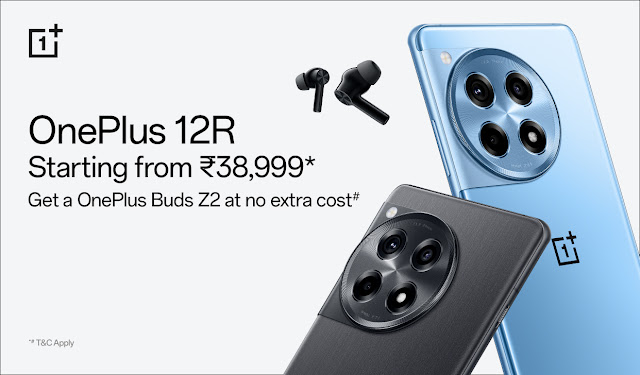
CONDITIONAL CONTROL STRUCTURES
Conditional Structure, also called Selection Construct, is used when certain task (group of program statements) has
to be executed for a particular situation/condition.
The condition is an expression that is being evaluated and is checked using comparison operators like ==, != , < , > … etc. For example:
There are 3 types of
conditional statements:
(a) if statement, if…else statement
(b) switch statement
(c) Ternary Operator ?: (you have already
learned this in chapter3)
Lets look at them in detail.
(a) if statement,
if…else statement
if statement is used when
we need to check one condition and provide one solution if the condition is True. Syntax is:
if (condition)
statement;
If the condition is TRUE, statement is executed, else program control
shifts to next line of code after the statement. For example,
if (x == 100)
cout
<< “x is Hundred”;
In
multiple statements, use curly braces { }. For example,
if (x == 100)
{
cout
<< “x is 100“;
cout << “x
is Hundred”;
}
if…else statement is used when we need to
check one condition and provide two solutions, one for True and another for the
False situation.
Syntax
is:
if (condition)
statement_1;
else
statement_2;
If condition is TRUE, goto statement_1 else goto statement_2.
if (x == 100)
cout << “x is 100”;
else
cout << “x is not 100”;
if…else statement (nested condition) is used
to check more than one condition and provide two or more solutions for the
different conditions.
if…else structure can be connected with more if…else structures
by placing one if…else block within another, by following a
proper sequence. This is the process of nesting in conditional statements.
An 'if' condition within another 'if' condition is called the nested if structure.
Syntax is:
if (condition1)
if
(condition2)
statement_1;
else
statement_2;
else
if
(condition3)
statement_3;
else
statement_4;
….. …..
….. …..
Following example demonstrates the use of nested if…else and determines if the value stored in variable x is positive, negative or zero.
if (x > 0)
cout << “x is positive”;
else
if (x < 0)
cout << “x is negative”;
else
cout << “x is 0”;
For multiple statements we must group them in blocks by
enclosing them in braces { }. For
example,
if (x > 0)
{
cout << “x is positive”;
cout << “x will
add”;
}
else
if (x < 0)
{
cout << “x is negative”;
cout<< “x will subtract”;
}
else
cout << “x is 0”;
PROGRAM 18: Find the biggest
among three numbers.
#include<iostream>
using namespace std;
int main()
{
int
a,b,c;
cout<<"Enter three numbers: ";
cin>>a>>b>>c;
if(a>b)
if(a>c)
cout<<"Biggest is: "<<a;
else
cout<<"Biggest is: "<<c;
else
if(b>c)
cout<<"Biggest is:
"<<b;
else
cout<<"Biggest is: "<<c;
}
|
Output
Enter three
numbers : 10 50 30
Biggest is: 50
|
(b) switch statement
The switch statement is used to check multiple conditions that are represented
by a case constant. When a specific case is satisfied, the lines of program code
within that case block is executed. Syntax is:
switch
(expression)
{
case
constant1:
group of
statements1;
break;
case
constant2:
group of
statements2;
break;
...
...
default:
default group
of statements;
}
An expression
in the switch statement holds a value
that will be compared to each case declaration.
If value
of expression is equal to case
constant1, then the lines of program code until
next case constant is executed. Every case usually ends with the break statement. A break statement terminates execution of remaining lines of code
within the current conditional/looping block and comes out of the switch
statement.
If expression was not equal to constant1, it will be checked against constant2
and so on. Finally, if the value of expression did not match any of the declared
constants, statements included within the default
label would be executed and then program-control would exit the switch statement.
In the example given below, if value of x is 1,2,3 the display will be “x is below 4”, otherwise the output display will be “x is above 3”.
switch (x)
{
case 1:
case 2:
case 3:
cout << “x is below 4”;
break;
default:
cout << “x is above 3”;
}
PROGRAM 19: Write a program to calculate the area of a square, rectangle,
circle and triangle using the switch statement. Assume scale in
centimeters.
#include<iostream>
using namespace std;
main()
{
int
a,b,c; int s;
float area;
cout<<"Square [1]\n";
cout<<"Rectangle [2]\n";
cout<<"Circle [3]\n";
cout<<"Triangle [4]\n";
cout<<"Enter your choice (1, 2, 3, 4) : ";
cin>>s;
cout<<"\n";
switch(s)
{
case 1:
cout<<"Enter Length: "; cin>>a;
area=a*a; // area of
square = side x side
cout<<"\nArea of the Square is:
"<<area<<" sq.cm";
break;
case 2:
cout<<"Enter Length : ";
cin>>a;
cout<<"Enter Breadth: "; cin>>b;
area=a*b; // area of
rectangle = length x breadth
cout<<"\nArea of the Rectangle is:
"<<area<<" sq.cm";
break;
case 3:
cout<<"Enter Radius: ";
cin>>a;
area=float(3.14*a*a); // area of
circle = pi x radius x radius
cout<<"\nArea of the Circle is:
"<<area<<" sq.cm";
break;
case 4:
cout<<"Enter Base Length : "; cin>>a;
cout<<"Enter Height
: "; cin>>b;
area=float(0.5*a*b); // area of
triangle = 1/2 x base x height
cout<<"\nArea of the Triangle is:
"<<area<<" sq.cm";
break;
default:
cout<<"\nInput Not in Range ...
Please enter numbers 1 / 2 / 3 / 4 ";
}
}
|
Output
(c) Conditional Ternary Operator
The Conditional Ternary Operator represented by the symbols ?: is used as a conditional statement where one condition can be checked and two possible solutions can be provided.
Syntax is:
condition
? if true,
solution1 : if false, solution2
If condition is satisfied
(true) then solution1 is executed, otherwise solution2
is executed. For example, in the
following ternary statement, if a is greater than b, then system displays “a is
Big” else “b is Big” gets displayed.
a>b ? cout<<”a is
Big” : cout<<”b is
Big”;
PROGRAM 20: Check if a number is ODD or EVEN using ternary operator.
#include<iostream>
main ()
{
int a;
std::cout<<"Enter
a Number "; std::cin>>a;
std::cout<<a<<(a%2==0 ? "
is EVEN" : " is ODD");
}
|
Output
LOOPING (ITERATION) STRUCTURES
Whenever we need to repeatedly perform a task until
a specific condition is met, we can use the looping/iteration
structures. There are 3 types of iteration structures: while loop, do…while
loop and for loop.
Note: Iteration means repetitive process. When creating an iteration loop, we must always consider that the loop has to end at some point, therefore we must provide some method to force the condition to become false at some point, otherwise the loop will continue executing the code forever (press CONTROL+C to stop an infinite loop).
(a) while loop is an entry controlled block of
statements that are repeatedly executed
until the given condition is not satisfied. Syntax is:
while (expression)
{
statement; modifier;
}
At first, the expression (condition) is checked and if it is TRUE, statement or statements within the while loop are executed, which includes the modifier. A modifier brings change to the condition. The control then goes back to the top of loop and expression is checked once again. The process (loop) continues until the expression goes FALSE.
For Example:
while(a < 3)
{
cout<<”This
is while loop”; a++;
}
PROGRAM 21:
Using while
loop, print the first n natural numbers.
#include <iostream>
using namespace std;
main ()
{
int i=1, n;
cout << "Enter a number: "; cin >> n;
cout<<"First
"<<n<<" natural numbers are";
while (i<=n)
{
cout << ", "<< i++ ;
}
}
|
Output
Enter a number: 5
First 5 natural numbers are, 1, 2, 3, 4, 5
|
(b) do … while loop is an exit controlled block of
statements that are repeatedly executed
until a given condition is not satisfied. Here, the statements are executed atleast
once. Syntax is:
do
{
statement; modifier;
}
while (condition);
In do while loop, the statements and modifier are executed
first, and then the condition is checked. If condition is TRUE, the loop is executed once again. This loop continues until the condition goes FALSE. E.g:
do
{
cout<<”This
is do while loop”; a++;
}
while (a<3);
PROGRAM 22: Using do while loop,
print the first n whole numbers.
#include <iostream> using namespace std; main () { int i=0, n; cout << "Enter a number: "; cin >> n; cout<<"First "<<n<<" whole numbers are"; do { cout << ", "<< i++ ; } while (i<n); }
|
Output
Enter a number: 5
First
5 whole numbers are, 0, 1, 2, 3, 4
|
(c) for loop is an entry controlled block of statements that are repeatedly executed until a given
condition is not satisfied. The for loop header has a control expression
which defines 3 parts: initializer,
condition-checker and modifier. It is followed by for loop footer which is the statement
block. Syntax is:
for
(initialization; condition; modifier)
{
statement;
}
Example of for loop:
for (i = 0; i<5; ++i)
cout<<”This is for loop”;
}
Functional Steps in ‘for’
Loop:
Step 1. Initialization
is the first step in for loop, where
one or more variables can be initialized with a starting value. This part is
executed only once. Eg: i = 1;
Step 2. Condition is checked in
the second step of for loop. If condition
is true, control shifts to step 3, otherwise for loop ends and control jumps out of the loop. Example of
condition: i < 10;
Step 3. Statement or a block of instructions
is executed in step 3 of for loop. It
can either be a single statement or a block of statements enclosed in braces { }.
Step 4. Modifier brings changes that effect the looping condition. It makes changes to initialized/other variables usually by increment or decrement
operation, after which control shifts back to Step 2 and the
process of condition checking repeats, followed by steps 3 and 4. Example of modifier: ++ i
PROGRAM 23: Using
for loop, print entire range of n integers.
(Eg: if n=2, print -2, -1, 0, 1, 2)
Note: An integer is a collection of negative and positive whole numbers along with zero.
#include <iostream>
using namespace std;
main ()
{
int
i=0, n;
cout << "Enter a number: "; cin
>> n;
cout << "Integers Upto
"<<n<<" are";
for
(i=-n; i<=n; ++i)
{
cout << ", "<< i ;
}
}
|
Output
Enter a number: 3
Integers Upto 3 are, -3, -2, -1, 0, 1, 2, 3
|
"for" Loop Without Control Expressions:
In a for loop, the initialization,
condition and modifier expressions can remain empty, and separated by semicolon.
E.g.1 : for( ; n<10 ; n++)
It specifies no initialization, but there is a modifier.
Here, the variable n must have been initialized before for loop starts.
E.g.2 : for( ; n<10 ; )
It specifies no initialization and no modifier.
E.g.3 : for(;;);
It is an infinite loop and system hangs (press “Control C” to exit loop).
"for" Loop With Control Expressions Separated By Comma Operator:
The comma operator (,)
can be used to specify more than one expression within the 3 control
expressions of the for loop.
E.g. for (int n=0, i=100 ;
n!=i ; n++, i-- ) { cout<<n<<" ";};
It executes 50 times and prints from 0 to 49.
The loop stops executing when n=i=50
Brain Teaser
Discuss
result of this for loop with valid reasoning.
for (int n=1, i=100 ; n!=i ; n++, i-- )
{ cout<<n<<" ";}
|
JUMP STATEMENTS (UNCONDITIONAL CONTROL STRUCTURES)
The Jump Statement is used to come out of program
blocks, conditional statements or the iteration structures. It is also called “unconditional
control structure” because it can be used in any part of the program, without
checking for any condition. We are covering four common jump statements: break, continue, goto,
return
(a) The break Statement
break is an unconditional control statement that is used to terminate from a program block. It forces termination of a loop
or the switch structure. Syntax
is:
break;
PROGRAM 24: Write a program using break statement in a loop, to accept
multiples of 10 (eg: 10, 120, 700…) and exit as soon as it’s not multiple of 10.
#include<iostream>
using
namespace std;
main()
{
int n=1;
while (n)
{
cout<< "Enter Multiples of Ten:
"; cin>>n;
if (n%10)
{
cout<<n<<" is not multiple
of 10 ... goodbye";
break;
}
}
}
|
Note: while(n) means, whenever the value of n is
TRUE (not zero), execute statements given inside the while loop. Remember that
FALSE=0 and TRUE≠0. Since at the beginning n=1, while(n) becomes TRUE, and loop executes. Then control enters “if condition”, where value of n%10 is checked. When
n%10=0 (n is a multiple of 10) the “if
condition” is not executed, otherwise “if
condition“ is executed and the loop breaks.
Output
Brain Teaser: Discuss result of this program and the reason.
Note: while ( !n ) reads while NOT n or, while n is not TRUE.
#include<iostream>
using namespace std;
main()
{
int
n;
while ( !n )
{
cout<< "Enter Multiples of Ten: ";
cin>>n;
if
(n%10)
{
cout<<n<<" is not multiple of 10 ...
goodbye";
break;
}
}
}
|
(b) The continue
Statement
continue is an unconditional control statement that is used to skip rest
of the instructions within a loop and proceed with next iteration. It
forces the next iteration in a loop. Syntax is,
continue;
PROGRAM 25: Using any “loop” and “continue statement” print all numbers
from 10 to 1, except 8, 5, 2.
#include <iostream>
using namespace std;
main ()
{
for (int n=10; n>0; n--)
{
if (n==8 || n==5 || n==2)
continue;
cout << n << ", ";
}
}
|
Output
(c) The goto Statement
goto is an unconditional control statement that
is used to jump to a specified point within the program code. The destination point is identified by a
label, which is used as an argument with goto statement. A label is a valid identifier that is declared by label name and a colon
symbol “:”.
Syntax of goto is: goto label;
e.g.
goto point1;
PROGRAM 26: Print even
numbers within first n natural numbers using goto statement.
#include <iostream>
using namespace std;
main ()
{
int n,i;
cout<<"Enter final range value: ";
cin>>n;
cout<<"Even numbers from 0 to
" << n << " are: ";
point1:
if(i%2==0)
cout<<i<<" ";
i++;
if
(n>=i)
goto point1;
}
|
Note: Declaration
int n,i would assign the value 0 to both n and i by default.
Output
Enter final range value: 7
Even numbers from 0 to 7 are: 0 2 4 6
|
(d) The return
Statement
return is an unconditional control statement used in a function to terminate the execution of code within that function and then return back to its origin (the calling function). Remember that C++ programs start with the main() (or the main function). A function can have any number of return statements.
Syntax is: return(value);
Example : return(0);
Note: Please refer to
Chapter1, Program1, Method1. You will learn more on functions in the upcoming chapters.
PROGRAM 27: Print the first n multiples of 4.
#include <iostream>
using namespace std;
main ()
{
int n,count;
cout<<"Enter any number: ";
cin>>n;
cout<<"First "<<n<<" multiples of 4 are: ";
for (int i=1 ; i<=n ; i++)
cout<<4*i<<" ";
}
|
Enter any number: 3
First 3 multiples of 4 are: 4 8 12
|
PROGRAM 28: Find the factorial of a number.
#include <iostream>
using namespace std;
main ()
{
int n, f=1;
cout<<"Enter any number: "; cin>>n;
for (int i=1 ; i<=n ; f*=i, i++);
cout<<"Factorial of "<<n<<" is: "<<f;
}
|
Output
Enter any number: 5
Factorial of 5 is: 120
|
PROGRAM 29: Check a Prime number without any iteration statement.
Hint: use ‘goto’ statement.
(A prime number cannot be –ve, 0 or 1. First prime number is 2)
#include <iostream>
using namespace std;
int main ()
{
int p, n=2;
cout<<"Enter any number: "; cin>>p;
a1:
if (!(p>1))
{
cout<<"Enter another number: "; cin>>p;
goto a1;
}
a2:
if(p!=2 && p%n==0)
{
cout<<p<<" is Not Prime Number";
return(0);
}
n++;
if (n<=p/2)
goto a2;
cout<<p<<" is a Prime Number";
}
|
Output
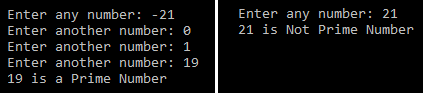
PROGRAM 30: Print all Prime numbers within n natural numbers.
#include <iostream>
using namespace std;
int main ()
{
int n;
cout<<"Enter any number: "; cin>>n;
while(n<=1)
{
cout<<"Enter another number: "; cin>>n;
}
cout<<"Prime numbers upto "<<n<<" are: ";
for (int i=2, j; i<=n; ++i)
{
j=2;
while( j<=i/j )
{
if (! ( i%j ) )
break;
j++;
}
if ( j>i/j )
cout<<i<<" ";
}
}
|
OUTPUT
CHAPTER EVALUATION EXERCISE
A) Choose the most appropriate
answer (1 mark)
1. _____________ statements control the order in
which instructions are executed.
(a)
conditional (b) un-conditional (c)
looping (d) a and b
2.
switch statement is a _____________ control structure.
(a)
conditional (b) un-conditional (c)
looping (d) a and b
3.
_____________ satisfies the following conditions:
if true, check another condition with
alternative and,
if false, give alternative.
(a) if-else (b)
if-else...if (c)
if...if-else...else (d) if-else...if-else
4.
_____________ is the biggest, if following condition is True: (p>q<r)
(a)
p (b) q (c) r (d) none
5.
A 'break' control can be used in _____________ statements.
(a) if-else (b)
loop, switch (c) ternary (d) a and b
6.
_____________ structure is an exit controlled loop.
(a) while (b)
do-while (c) for (d) a and b
7.
The _____________ of 'for' loop is executed only once.
(a)
initializer (b) condition (c)
modifier (d) program statements
8.
goto is a _____________ statement.
(a)
conditional (b) un-conditional (c) jump (d)
b and c
9.
_____________ skips rest of instructions within a loop and proceeds with next
iteration.
(a)
continue (b) break (c) goto (d)
return
10.
_____________ is the output of below given 'for' loop:
for (i=1, j=1 ; i<4 ;
(j<3?cout<<i*j<<"\n":cout<<"---\n"),
++j, ++i);
(a) 1 (b)
1 (c) 1 (d) 4
4 3 3 ---
--- 4 ---
---
ANSWERS
1)
d 2) a 3)
c 4) c 5)
b 6) b
7) a 8) d 9) a 10)
a
B) Fill in the blanks (1 mark)
1.
____________ statement controls the repetition of executing program statements.
2.
A ____________ is evaluated and checked using comparison operators.
3.
for(;;) has ____________control expressions.
4.
If i=1, then while(!i) is True or False?
5.
____________ terminates execution of code within a function and gets back to
its calling function.
6.
In 'for' loop, after a modifier is executed, control shifts to ____________
7.
In ____________ loop, the statements are
executed atleast once.
8.
What is the output of this statement (Hint: Think ASCII code).
'a'>'A'?
cout<<"Yes":cout<<"No";
9.
An 'if' condition within another 'if' condition is called ____________
10.
In 'for' loop, after execution of program instructions, the control shifts to
____________
ANSWERS
1) Looping 2) condition 3) no 4) True 5) return()
6) condition-checker 7)
do-while 8) Yes 9) nested if
10)
modifier
C) State whether True or False
(1 mark)
1.
Several instructions grouped in a block are compound statements.
2.
Selection construct is an un-conditional control structure.
3.
In 'switch' statement, if no 'case' is satisfied, then statements in default
gets executed.
4.
?: is an unconditional operator.
5.
for loop has a header and footer.
ANSWERS
1)
True 2) False 3) True 4) False 5)
True
D) Short answer questions (5
marks)
1.
(i) What would be the output of this program:
int
a;
for( ; ; )
{
if(!a)
{
cout<<"Inside IF: Exiting Loop";
break;
}
cout<<"Inside FOR: Infinite Loop" ;
}
(ii)
What would be above output if a=1 at declaration ?
2.
What are the functional steps in a FOR loop.
3.
Briefly explain 'for' loop, with & without control expressions.
E) Subjective questions (10
marks)
1. Explain goto statement with an example.
2. What is the difference between a while loop
and do-while loop?